Introduction: Why Python?
What is Python?: A brief introduction to Python, its history, and why it’s one of the most popular programming languages today.
Why Learn Python?: Exploring the reasons why Python is an excellent language for beginners and experienced developers, from its simplicity to its versatility in various fields like data science, web development, and automation.
Python’s Key Features: An overview of Python’s main features such as readability, scalability, and vast library support.
Chapter 1: Getting Started with Python
Setting Up Python: How to install Python on Windows, macOS, and Linux, and setting up a development environment (e.g., Anaconda, Jupyter Notebook, VSCode).
Your First Python Program: Writing your first Python script with “Hello, World!” and understanding the basic structure of Python code.
Understanding Python Syntax: Introduction to Python syntax, indentation, and how it differs from other programming languages.
Using Python Interactive Mode: Learning how to use the Python shell for quick testing and exploration of code.
Chapter 2: Variables, Data Types, and Operators
Variables in Python: Defining variables, naming conventions, and understanding the concept of dynamic typing in Python.
Python Data Types: Introduction to basic data types such as integers, floats, strings, lists, tuples, dictionaries, and sets.
Type Conversion: How to convert between data types (e.g., from string to integer).
Operators: Understanding arithmetic, comparison, and logical operators in Python.
Chapter 3: Control Flow and Functions
Conditionals: How to use if, elif, and else statements to control the flow of your Python programs.
Loops: Introduction to for and while loops, iterating over different data structures, and using break and continue statements.
Functions: Defining and calling functions, understanding function arguments, return values, and scope.
Lambda Functions: Introduction to anonymous functions and their uses in Python.
Error Handling: Using try, except, finally to handle exceptions and ensure your code runs smoothly.
Chapter 4: Data Structures in Python
Lists: How to use lists, list slicing, and list methods for manipulating data.
Tuples: Understanding immutable data types and when to use tuples.
Dictionaries: Using key-value pairs for efficient data storage and retrieval.
Sets: Introduction to sets and their importance in eliminating duplicate items.
Comprehensions: List comprehensions, dictionary comprehensions, and set comprehensions for more compact and readable code.
Chapter 5: Object-Oriented Programming (OOP) in Python
Classes and Objects: Understanding the basics of object-oriented programming, creating classes, and defining objects.
Attributes and Methods: How to define attributes (variables) and methods (functions) within classes.
Inheritance: How to inherit properties and methods from other classes to create a class hierarchy.
Polymorphism and Encapsulation: Using polymorphism (methods with the same name) and encapsulation (hiding data).
Magic Methods: Introduction to special methods like init, str, repr, and more.
Chapter 6: Working with External Libraries
Standard Library: Exploring Python’s standard library and its built-in modules such as math, os, sys, datetime, and json.
Third-Party Libraries: How to install third-party libraries using pip and virtualenv. Popular libraries such as requests, numpy, pandas, and matplotlib.
Package Management: How to manage dependencies in your Python projects using pip and requirements.txt.
Chapter 7: File Handling and Data Persistence
Reading and Writing Files: How to read from and write to files using Python, including text and binary files.
Working with CSV Files: How to work with CSV files using the csv module for storing tabular data.
Working with JSON: Using Python’s json module to read and write JSON files for data storage and communication.
Databases in Python: Introduction to connecting Python with databases such as SQLite, MySQL, and PostgreSQL.
Chapter 8: Advanced Python Concepts
Decorators: Understanding how decorators work to modify the behavior of functions or methods.
Generators and Iterators: Introduction to generators and the yield keyword for creating iterators in Python.
Context Managers: Using with statements to manage resources like file handling and database connections.
Multithreading and Multiprocessing: Basic concepts of parallelism, threads, and processes for making Python programs more efficient.
Chapter 9: Working with Data in Python
Data Analysis with Pandas: Introduction to the pandas library for data manipulation, cleaning, and analysis.
Visualization with Matplotlib: Creating simple plots and visualizations using matplotlib and seaborn.
NumPy for Numerical Computations: Introduction to numpy for working with arrays, matrices, and performing numerical computations.
Data Wrangling and Cleaning: Techniques for dealing with messy or missing data using Python libraries.
Chapter 10: Building Projects with Python
Creating a Web Scraper: How to use requests and BeautifulSoup to scrape data from websites.
Building a Web Application with Flask: Introduction to building web apps with the Flask framework.
Creating a Simple Command-Line Tool: How to build a basic command-line tool using Python’s argparse module.
Unit Testing in Python: Writing test cases with Python’s unittest module to ensure code reliability.
Chapter 11: Debugging and Optimizing Python Code
Using the Debugger: Introduction to debugging with Python’s built-in pdb debugger.
Profiling and Performance: Techniques for optimizing Python code and measuring performance using cProfile and other profiling tools.
Refactoring Code: Best practices for writing clean, readable, and maintainable code.
Chapter 12: The Future of Python
Python 3.x vs. Python 2.x: Understanding the differences and why Python 3 is the future.
Emerging Trends: Exploring the latest trends in Python, such as machine learning, artificial intelligence, and the rise of Python for scientific computing.
Python Communities: How to get involved with Python communities and open-source contributions.
Next Steps in Learning: Resources for continuing your Python journey, such as books, online courses, and Python documentation.
Mastering Python: A Comprehensive Guide for Beginners and Beyond
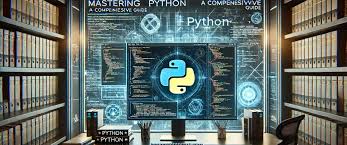